Apple Device Management overview #
Welcome to this set of articles on managing Apple devices in Applivery.
If you are not familiar with the Apple ecosystem, we recommend that you continue reading this introductory article that summarizes the main concepts that you must take into account to successfully deploy the management of your Apple devices.
Apple has a set of programs and solutions created to simplify and speed up the configuration of devices as well as the deployment of applications and content. If you’re already familiar with Apple Business Manager or programs like the Apple Device Enrollment Program (DEP), Apple Volume Purchase Program (VPP), and features like supervision, feel free to skip ahead. If not, let’s make a brief introduction:
Supported devices #
Apple Device Management support for Apple Devices covers all types of Apple devices:
- iPhones
- iPads
- MacBooks and iMacs
- Apple TV
- Apple Watch
Apple Business Manager #
Apple Business Manager is a web-based portal for IT administrators to deploy iPhone, iPad, iPod touch, Apple TV, and Mac all from one place. Working seamlessly with other MDM solutions like Applivery, Apple Business Manager makes it easy to automate device deployment, purchase apps and distribute content, and create Managed Apple IDs for employees.
The Device Enrollment Program (DEP) and the Volume Purchase Program (VPP) are completely integrated into Apple Business Manager, so organizations can bring together everything needed to deploy Apple devices.
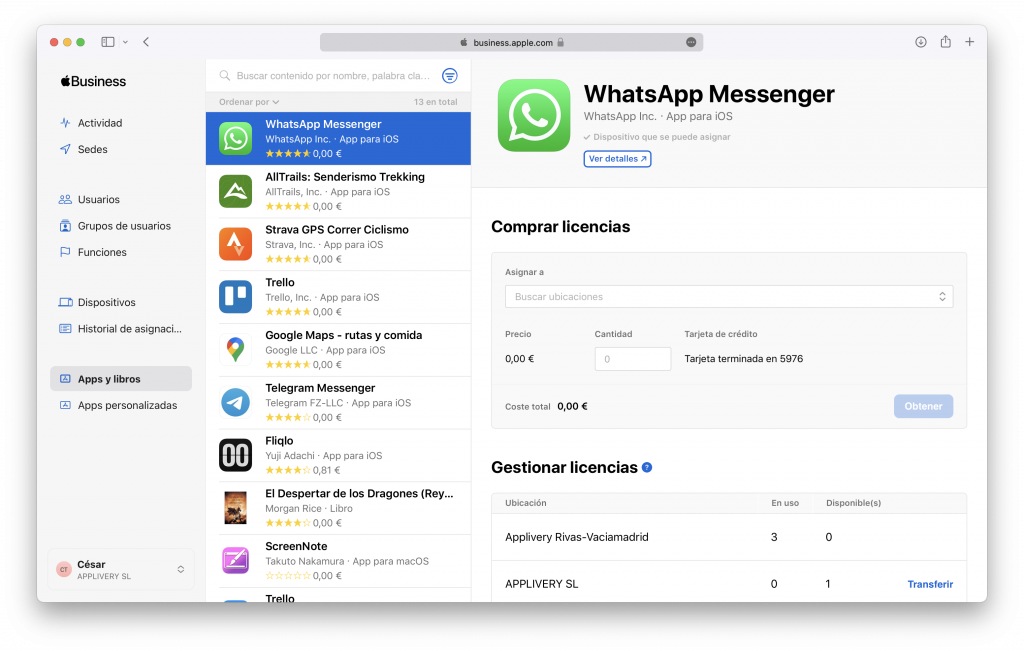
Device Enrollment Program (DEP) #
Apple Device Enrollment Program (also known as DEP) is a tool integrated with Apple Business Manager that allows organizations to fully automate the enrollment process of Apple Devices in MDM solutions like Applivery. In the beginning, it was focused on new devices but starting with iOS 11, now it supports enrolling already purchased devices as well. DEP helps organizations enable supervision, MDM enrollment, skip setup steps beside many other features. You can read more about Apple DEP here.
Volume Purchase Program (VPP) #
Other important concepts #
Supervision mode #
Apple Configurator app #
Apple provides two apps that will help you take advantage of the Apple Device Enrollment Program by registering your devices in your Apple Business Manager account.
- Apple Configurator (for Mac) is a MacOS app that makes it easy to deploy iPad, iPhone, iPod touch, and Apple TV devices in your school or business. It automatically registers your devices in Applivery and your Apple Business Manager (ABM) account.
- Apple Configurator (for iPhone) is an app that makes it easy to assign any Mac with the T2 Security Chip or Apple Silicon to your Apple Business Manager organization and manage it through Applivery.
You can read more about both apps here.
Next steps #
Now that the main concepts are a bit more clear, we suggest following the next steps:
- Determine whether you will need device supervision privileges.
- Select the enrollment method that best fits your feature requirements and deployment workflow.
- Plan your organization’s app deployment strategy.
- Choose whether or not to use Apple IDs in your deployment.
If you already have a strong grasp of the Apple Device Management concepts and would prefer to get started with your deployment straight away, feel free to refer to our quick-start guide here.